1. What is a Service Worker?
2. The man in the middle
3. The State of the Art
## What is a service worker?
It is an **event driven** worker that does not need any client to be alive,
it's **tied to its registration** only.
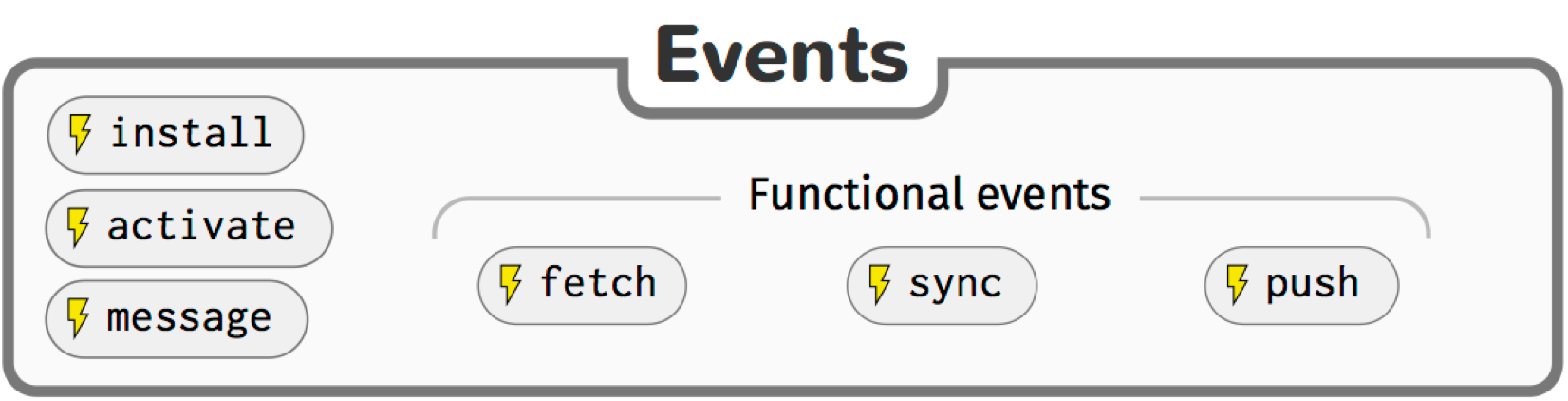
How to **register** a service worker?
```js
// index.js
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('path/to/sw.js', {
scope: 'path/to/scope/'
}).then(...);
}
```
How to handle **life cycle**?
```js
// service-worker.js
self.oninstall = function (event) {
event.waitUntil(setupCurrentWorker());
};
self.onactivate = function (event) {
event.waitUntil(teardownPreviousWorker());
};
self.onfetch = function (event) {
event.respondWith(fetch(event.request)); // pass through
}
```
[Complete life cycle](https://github.com/delapuente/service-workers-101/blob/master/sw101-letter-sA.svg)
How to know your **worker is ready**?
```js
// client.js
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('path/to/sw.js');
navigator.serviceWorker.ready.then(/* You know is ready! */);
}
```
### Service Worker 101
An [infographic on GitHub](https://github.com/delapuente/service-workers-101)
Your [copy for free](https://github.com/delapuente/service-workers-101/blob/master/sw101.png)
### More on Service Workers
Don't miss [latest changes on the specification](https://slightlyoff.github.io/ServiceWorker/spec/service_worker/index.html)
Follow [live discussions](https://github.com/slightlyoff/ServiceWorker/issues)
And [ask on stackoverflow](http://stackoverflow.com/search?q=service-workers)!
## The man in the middle
off-label uses of Service Workers
### The Service Worker Cookbook
> This cookbook contains dozens of practical, detailed, and working examples of service worker usage. These examples are for developers from beginner to expert and illustrate a number of APIs.
Take a [look](https://serviceworke.rs)!
### Interesting readings
* [Service Workers](https://hacks.mozilla.org/category/serviceworkers/) at Mozilla Hacks
* [Beyond offline](https://hacks.mozilla.org/2015/12/beyond-offline/)
* [Offline Recipes for Service Workers](https://hacks.mozilla.org/2015/11/offline-service-workers/)
* [Offline First](http://offlinefirst.org/)
### Cache from zip
More traditional use of service worker, it demonstrate an offline usage
but instead of caching every resource from its URL, it's caching all
the resources at the same time in a ZIP package.
[Demo](https://serviceworke.rs/cache-from-zip.html)
### API analytics
A direct application of a man in the middle. We use a service worker
to hijack the request, log some info and allow the request to complete.
[Demo](https://serviceworke.rs/api-analytics.html)
### Virtual Server
How about writing a REST API in the client side? It is possible with
Service Workers and a little help from
[ServiceWorkerWare](https://gihub.com/gaia-components/serviceworkerware)!
[Demo](https://serviceworke.rs/virtual-server.html)
### Render store
A lot of frameworks rely on template interpolation to render the model.
The NGA from Firefox OS introduces a kind of storage to store the interpolated
pages to [avoid interpolation delay](http://unoyunodiez.com/2015/09/07/modern-mobile-web-development-breaking-the-rules-beating-delays-improving-responsiveness-and-performance-ii/) upon a second request.
[Demo](http://localhost:8008)
### Dependency injection
Dependency injection is a technique that frees modules to create its dependencies.
Instead, modules declare the abstract APIs they will use and a service called _injector_
is used to provide the concrete implementations.
[Demo](https://serviceworke.rs/dependency-injector.html)
### Load balancer
In the mood of transparently replacing content, here is a load balancer implemented in
the client side.
[Demo](https://serviceworke.rs/load-balancer.html)
### Request deferrer
This technique withhold requests in a queue while offline to later recreate
the sequence of requests to the server once connectivity returns. It can be used
for agnostic back-end synchronization systems embedded in modern frameworks.
[Demo](http://localhost:3003/session-deferrer.html)
Browser support
- In Chrome? yes!
- And in Android Browser and Chrome for Android!
- In Nightly? yes!
- In Firefox? yes (since Firefox 44)!
\0/
### Where to check?
* [is ServiceWorker ready?](https://jakearchibald.github.io/isserviceworkerready/)
* [Platform Status](https://platform-status.mozilla.org/#service-worker)
* [Can I use ... ?](http://caniuse.com/)
### Major problems and workarounds
* ~~No storage on Firefox! Use an offline cache.~~
* ~~No `self.clients.claim()` implementation on Firefox! Use reload pattern.~~
* ~~No **dev tools** on Firefox! Use Chrome `:_(`~~
* Basic support of [Service Workers in dev tools](https://hacks.mozilla.org/2016/03/debugging-service-workers-and-push-with-firefox-devtools/). Use Chrome `:(`
### Tools & resources
* [serviceworke.rs](https://serviceworke.rs) for use cases & best practices
* [oghliner](https://github.com/mozilla/oghliner) to bootstrap a Service Worker enabled app.
* [ServiceWorkerWare](https://github.com/gaia-components/serviceworkerware) to ease Service Worker authoring.
* [offliner](https://github.com/delapuente/offliner) for adding a life cycle to your app.
* [sw-toolbox](https://github.com/GoogleChrome/sw-toolbox) another similar tool to ServiceWorkerWare.
* [upup](https://www.talater.com/upup/) a tool for _offlining_ your app.